Using Functions in Flows
When building a flow you can use the Function step to perform advanced formatting on data using JavaScript.
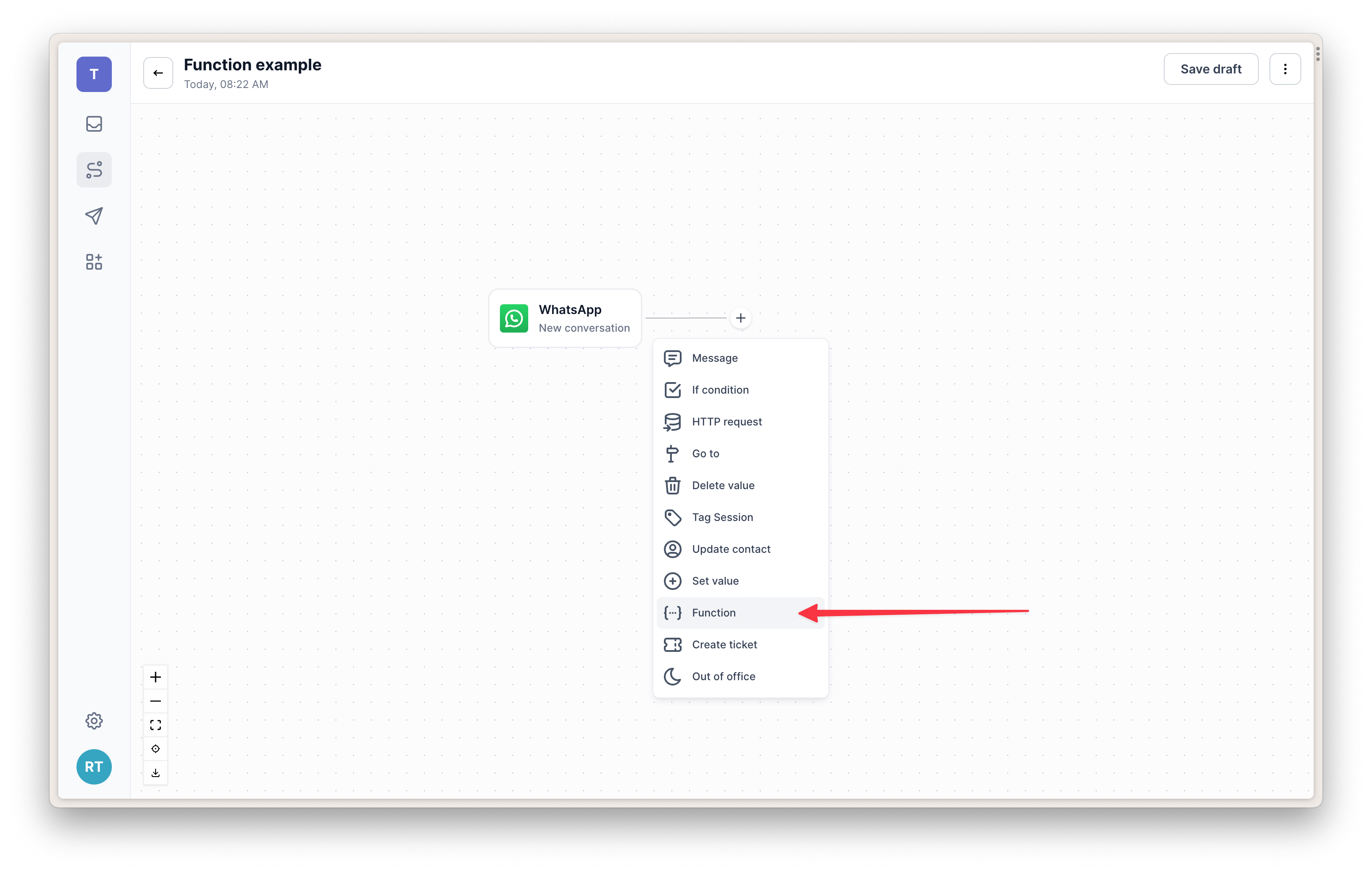
For example:
- extracting JSON from an HTTP response and converting it to a numbered list string that is inserted into a message
- changing dates into a preferred format
- formatting numbers to include decimals
- compiling a payment URL with various parameters
Input
Function steps have a required input field that expects a JSON object.
If you want to pass the response from an HTTP request step into a function as input you can do so by putting {{session.requestName.body}}
into the input field.
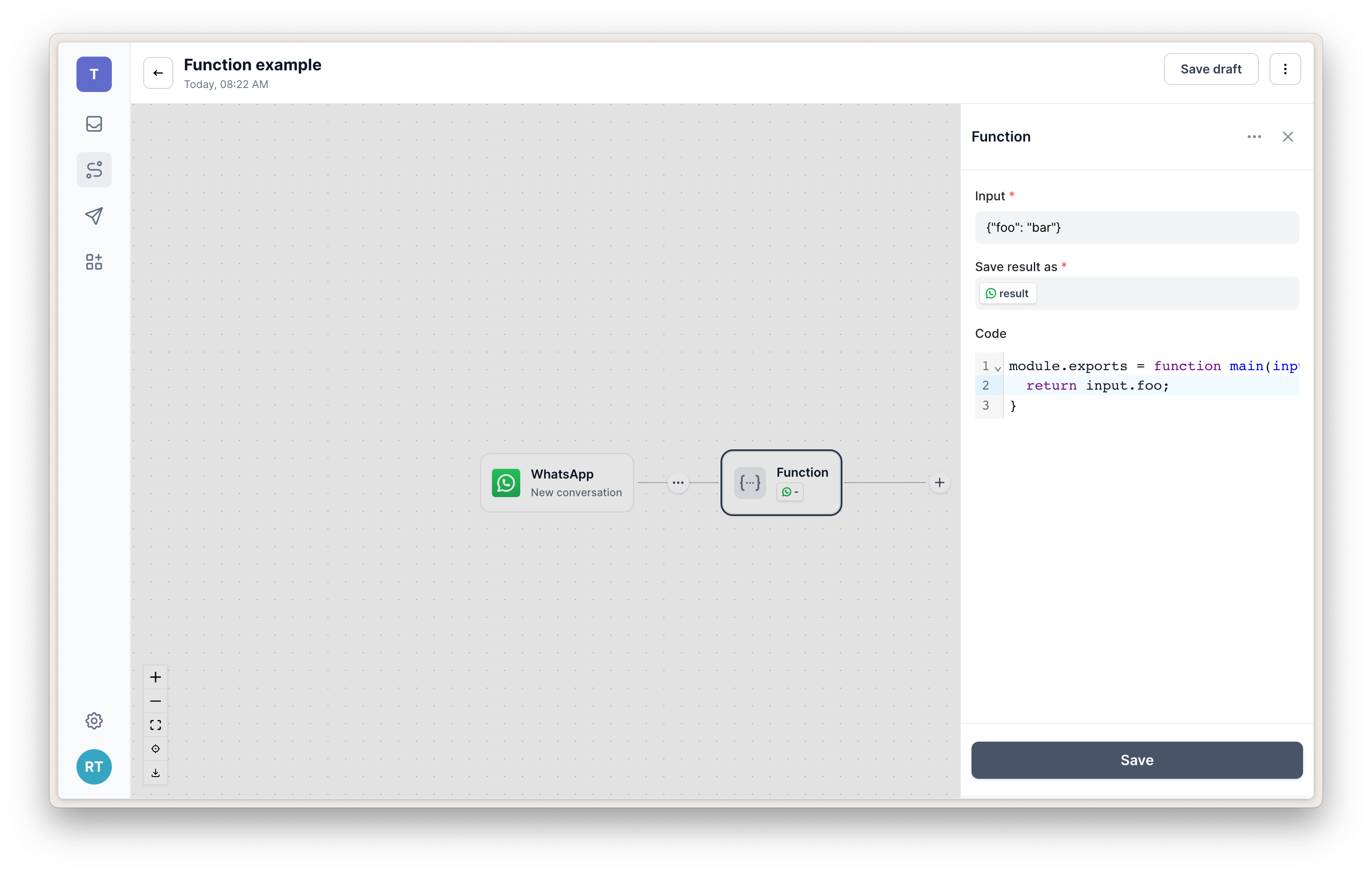
Tips for writing functions
The UI for editing functions is currently very minimal and it can be a bit tedious to continuously publish a new flow just to test if your function iteration is working.
We plan to introduce improvements to improve the code editing and testing experience but for now we recommend using a service like Stackblitz to write and test your functions first and then copy them over into the Function step code block.
For example:
// Test data
const data = {
accounts: [
"67388217",
"92037742",
"23099300"
]
}
// Function code
function main(input) {
var accountStrings = [];
for(var i = 0; i < input.accounts.length; i++) {
var accountNumber = input.accounts[i];
var accountString = `${i + 1}. ${accountNumber}`;
accountStrings.push(accountString);
}
return accountStrings.join("\n")
}
// Test function call
const result = main(data)
console.log(result)
// 1. 67388217
// 2. 92037742
// 3. 23099300
Also, we've found it helpful to use a tool like ChatGPT to write an initial function for you from a prompt but be careful to test and verify that it has given you a working function before inserting it into your flow.
Output
To access the output returned from your function you can use {{session.functionName.output}}
anywhere in your flow.
In a lot of cases it's best to return a fully formatted string of the text you want to display (e.g. an numbered list of n options) and then insert that output into a message.
If your function returns an object with multiple properties that you want to reference you will need to use the Set step to assign each of these to a custom session property. You cannot reference them using dot notation. For example, it is not possible to insert {{session.functionName.output.foo}}
into a message.
Caveats
- Functions cannot make network calls to the outside internet.
Planned improvements
We plan to introduce the following improvements in the near future:
- Larger code editing window
- Function testing within the UI
- A flow simulator so that you can test your flows from within the editing UI
- The ability to prompt AI to write a function for you